Arithmetic operators are used to perform mathematical operations in Python. They are fundamental to most programming tasks, from simple calculations to complex algorithms. This tutorial will guide you through the various arithmetic operators available in Python, including addition, subtraction, multiplication, division, modulus, exponentiation, and floor division.
Basic Arithmetic Operators
Python supports the following basic arithmetic operators:
- Addition (
+
) - Subtraction (
-
) - Multiplication (
*
) - Division (
/
) - Modulus (
%
) - Exponentiation (
**
) - Floor Division (
//
)
1. Addition (+
)
The addition operator adds two numbers.

2. Subtraction (-
)
The subtraction operator subtracts the second number from the first.
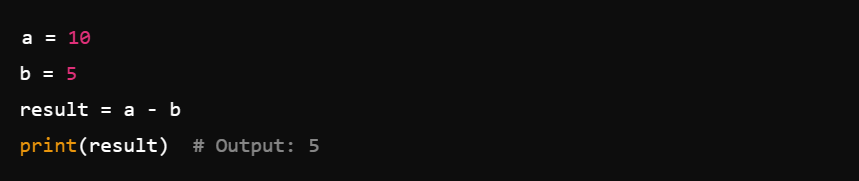
3. Multiplication (*
)
The multiplication operator multiplies two numbers.
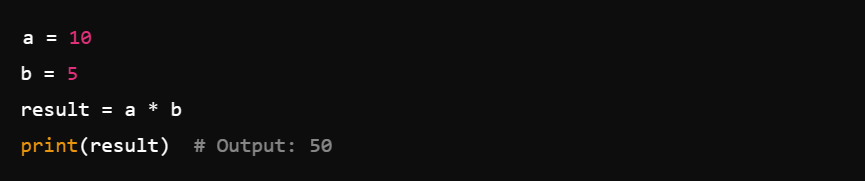
4. Division (/
)
The division operator divides the first number by the second. It always returns a float.
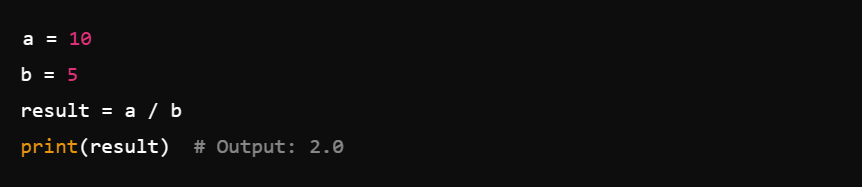
5. Modulus (%
)
The modulus operator returns the remainder of the division of the first number by the second.
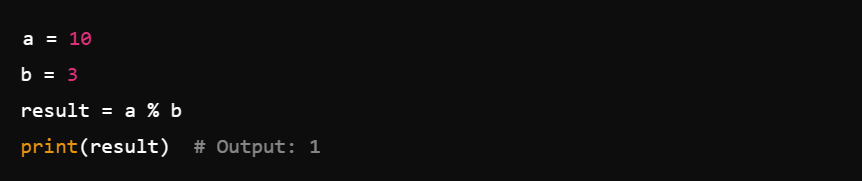
6. Exponentiation (**
)
The exponentiation operator raises the first number to the power of the second number.
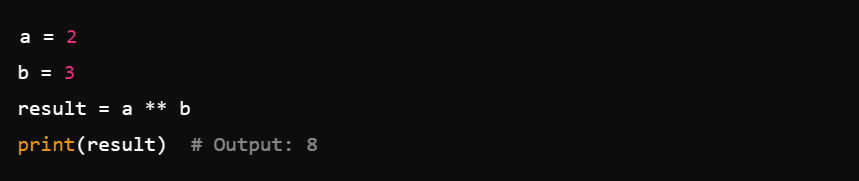
7. Floor Division (//
)
The floor division operator divides the first number by the second and then rounds down to the nearest whole number.
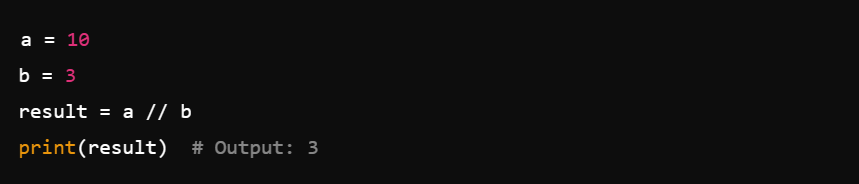
Combined Arithmetic Operators
Python also supports combined arithmetic operators, which combine an arithmetic operation with assignment. These include:
- Addition Assignment (
+=
) - Subtraction Assignment (
-=
) - Multiplication Assignment (
*=
) - Division Assignment (
/=
) - Modulus Assignment (
%=
) - Exponentiation Assignment (
**=
) - Floor Division Assignment (
//=
)
Example: Combined Arithmetic Operators
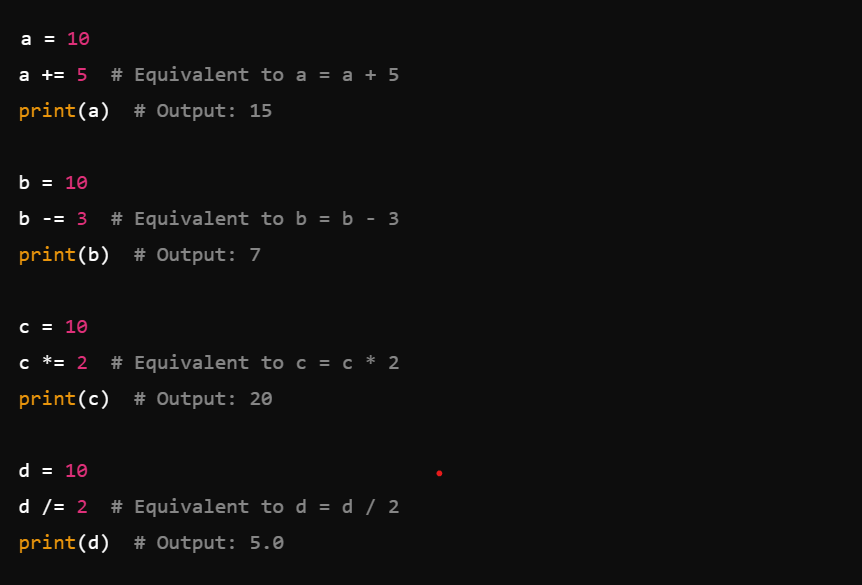
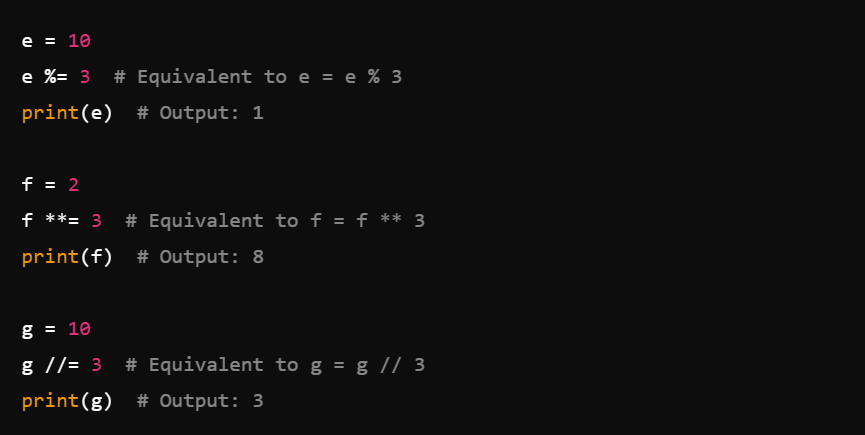
Order of Operations
When multiple operations are involved, Python follows the standard mathematical order of operations, also known as BODMAS/BIDMAS rules:
- Brackets
- Order (exponents, including roots, etc.)
- Division and Multiplication (left to right)
- Addition and Subtraction (left to right)
Example: Order of Operations

# Breaking it down:
# Brackets: (2 + 3) -> 5
# Order: 4 ** 2 -> 16
# Multiplication and Division (left to right): 5 * 16 -> 80; 80 / (6 – 2) -> 20.0