Sure! Let’s dive into a comprehensive tutorial on functions and modules in Python. We’ll cover the following topics in detail:
- Defining and Calling Functions
- Parameters and Return Values
- Built-in Functions
- Creating Custom Modules
1. Defining and Calling Functions
Defining a Function
In Python, you define a function using the def
keyword followed by the function name and parentheses. The function body contains the code that will be executed when the function is called.
Here’s the basic syntax:

Example

Calling a Function
To call a function, use its name followed by parentheses. If the function requires parameters, you pass them within the parentheses.

This will output:

2. Parameters and Return Values
Parameters
Functions can take parameters, allowing you to pass data into the function. These parameters are specified within the parentheses in the function definition.
Example

To call this function with an argument:

This will output:

Default Parameters
You can provide default values for parameters. If the caller doesn’t provide a value, the default is used.
Example

Calling the function without an argument uses the default value:

This will output:

Return Values
Functions can return values using the return
statement.
Example

You can capture the return value in a variable:

This will output:

Multiple Return Values
Python functions can return multiple values as a tuple.
Example

You can unpack the returned tuple into multiple variables:

This will output:

3. Built-in Functions
Python provides a rich set of built-in functions that are always available. Here are a few examples:
print()
: Prints to the console.len()
: Returns the length of a sequence.type()
: Returns the type of an object.sum()
: Returns the sum of a sequence.range()
: Generates a sequence of numbers.
Example
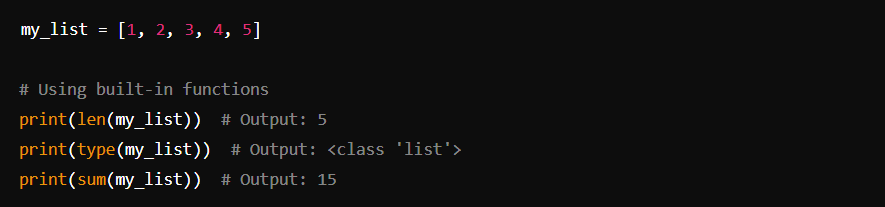
4. Creating Custom Modules
What is a Module?
A module is a file containing Python definitions and statements. The file name is the module name with the suffix .py
added. Modules help organize related functions and variables, making the code more modular and reusable.
Creating a Module
- Create a File: Create a new Python file (e.g.,
mymodule.py
).
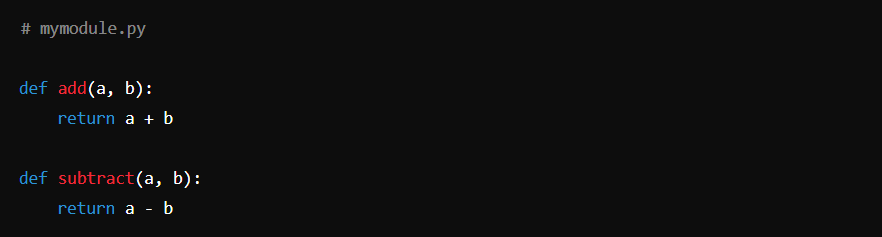
2. Using the Module: You can import and use this module in another Python file.
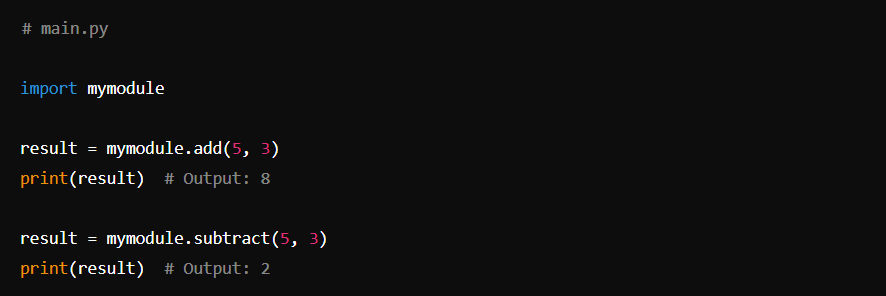
Importing Specific Functions
You can also import specific functions from a module.
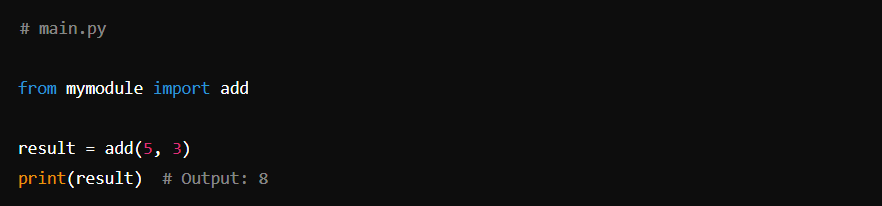
Aliasing Modules
You can provide an alias for a module to make it easier to use.
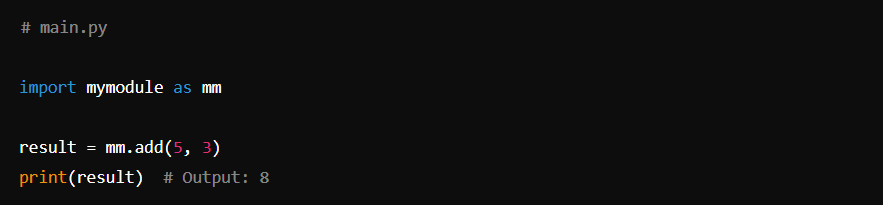
Creating Packages
A package is a way of organizing related modules into a directory hierarchy. A package must contain a special file named __init__.py
, which can be empty or contain package initialization code.
- Create the Package Structure:

2. Define Modules:
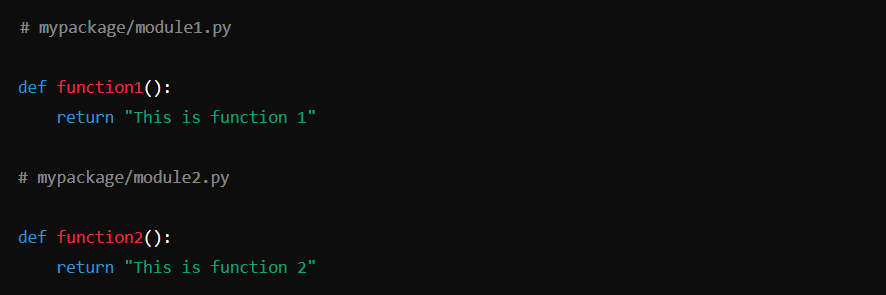
3. Use the Package:
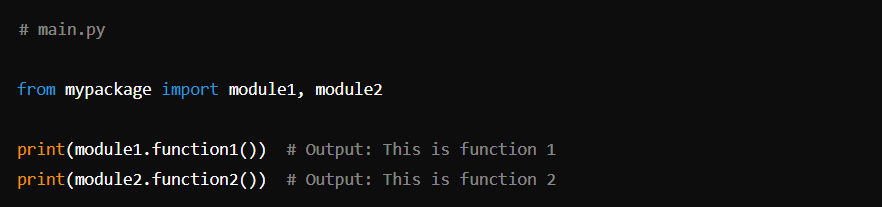
Importing from a Package
You can import specific functions from modules within a package.

Using __all__
You can control what is imported when a package is imported using the __all__
attribute in the __init__.py
file.

Now, when you import the package, only the specified modules will be imported.
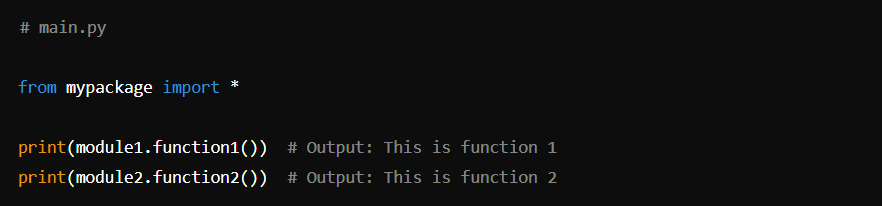
Conclusion
Understanding functions and modules in Python is crucial for writing efficient and modular code. Functions allow you to encapsulate code into reusable blocks, while modules and packages help you organize your code logically. This tutorial has covered the basics, but there’s always more to learn as you dive deeper into Python programming.