In this tutorial, we’ll explore two fundamental data structures in Python: Lists and Tuples. We’ll delve into how to create and modify lists, use various list methods, understand list slicing, and learn about tuples and their uses.
1. Lists
What is a List?
A list in Python is an ordered collection of items that can hold a variety of data types. Lists are mutable, meaning you can change their content without changing their identity.
Creating Lists
You can create a list by placing comma-separated values inside square brackets []
.
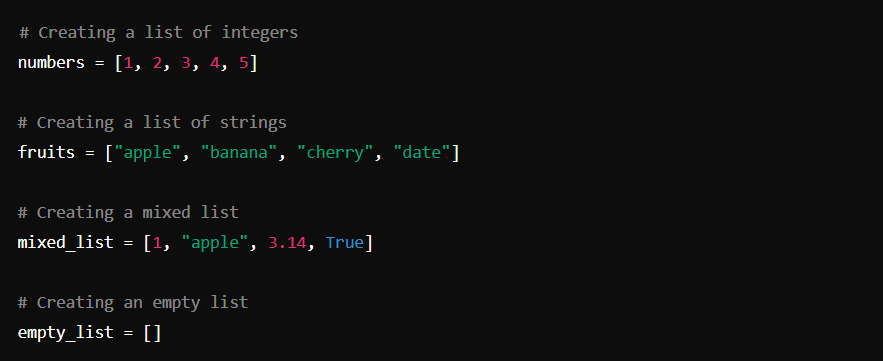
Modifying Lists
Since lists are mutable, you can change, add, and remove items after the list is created.
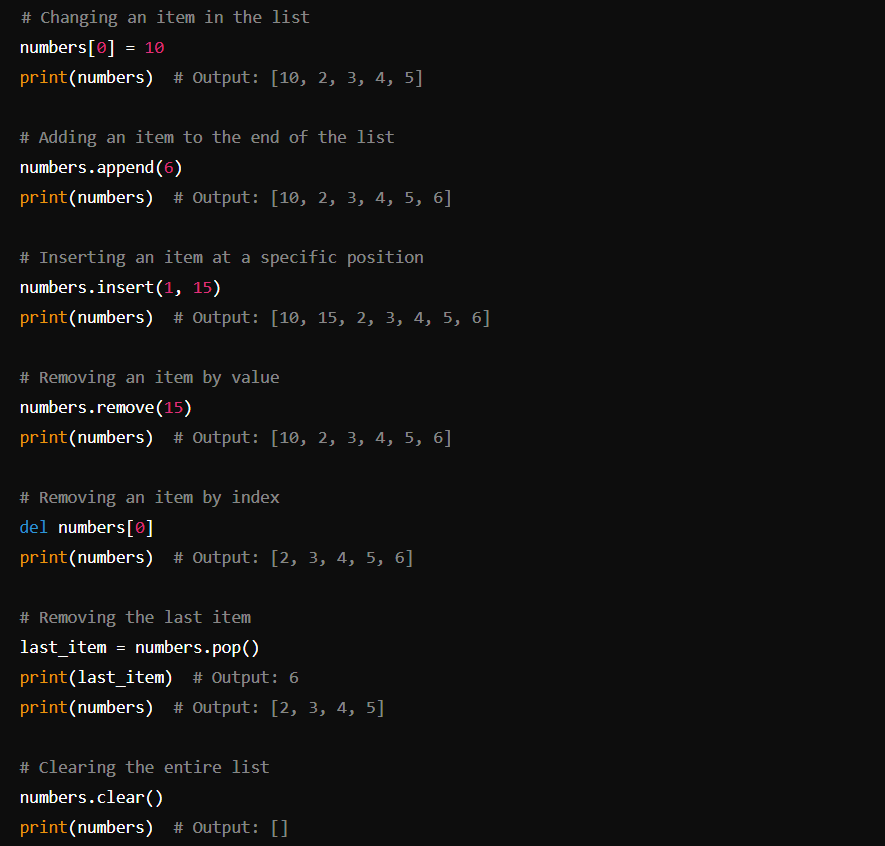
2. List Methods and Slicing
List Methods
Python provides various built-in methods to work with lists.
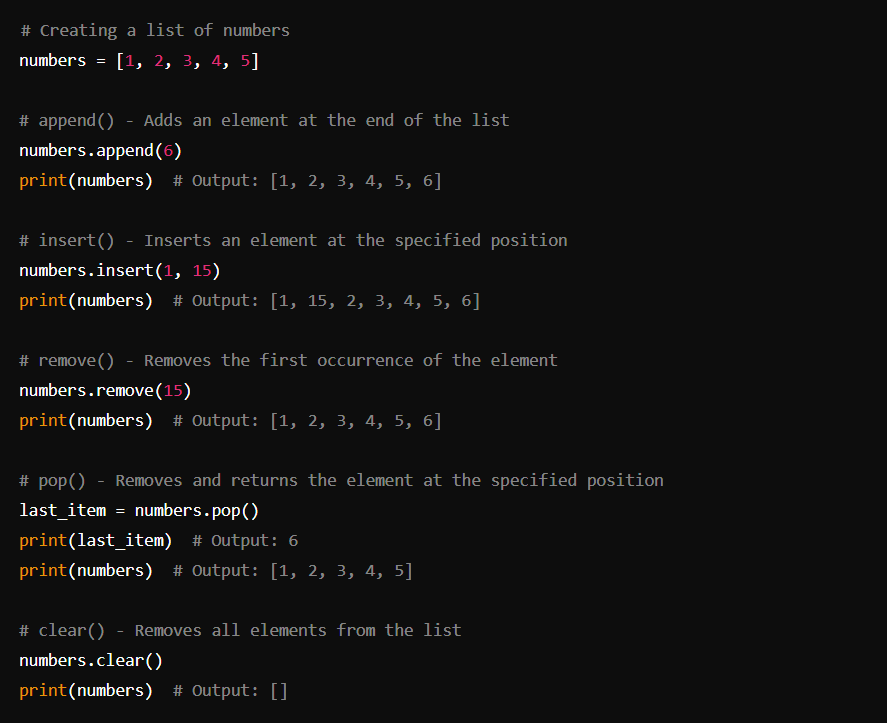
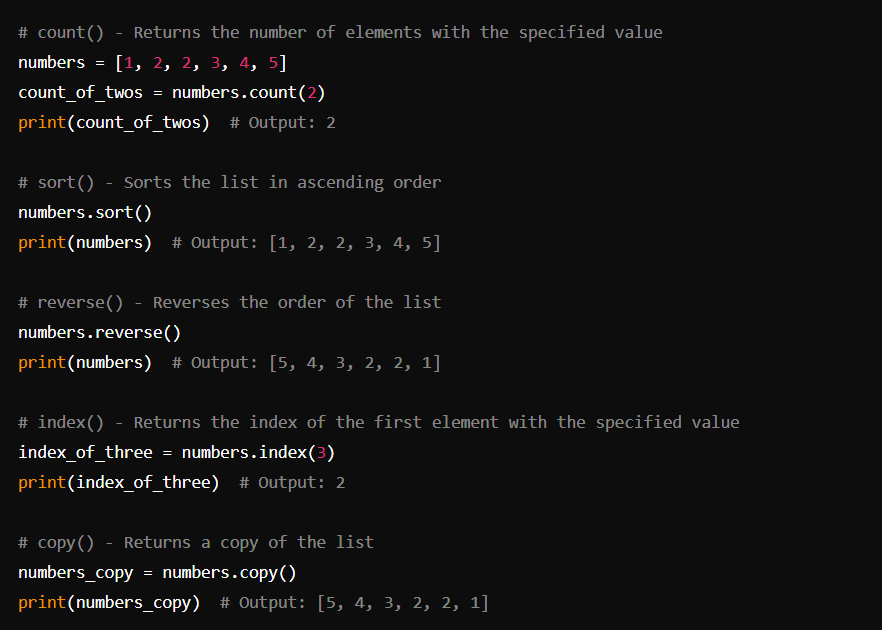
List Slicing
List slicing allows you to access a subset of the list. The syntax for slicing is list[start:end:step]
.

3. Tuples
What is a Tuple?
A tuple is an ordered collection of items similar to a list. However, tuples are immutable, meaning once a tuple is created, you cannot change its elements.
Creating Tuples
You can create a tuple by placing comma-separated values inside parentheses ()
.
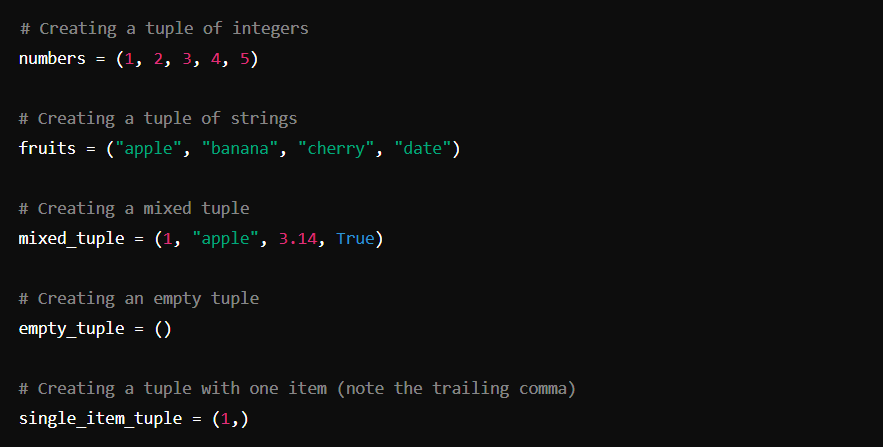
Accessing Tuple Elements
You can access tuple elements using indexing and slicing, similar to lists.
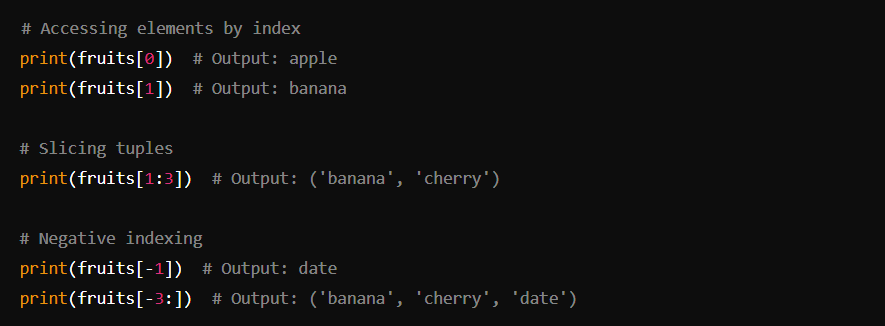
Tuples are Immutable
Since tuples are immutable, you cannot modify their elements.

Uses of Tuples
Tuples are useful when you want to ensure that the data remains constant. They are commonly used for fixed collections of items, such as coordinates, months of the year, or as keys in dictionaries.
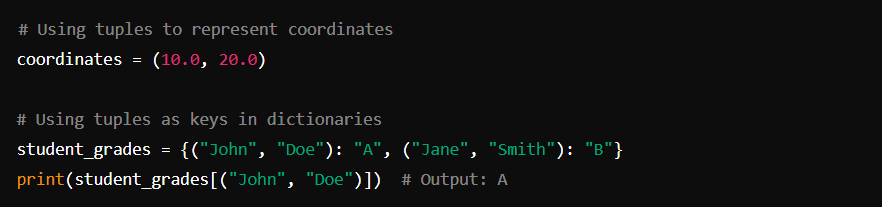
Conclusion
In this tutorial, we’ve covered the basics of lists and tuples in Python. We learned how to create and modify lists, explored various list methods and slicing techniques, and understood the immutability and uses of tuples. This foundational knowledge will be crucial as you dive deeper into more advanced data structures and algorithms in Python.