Control flow in Python allows you to direct the execution of your program based on conditions you specify. This guide will cover the fundamental concepts of control flow, including if
, elif
, and else
statements, nested conditions, and Boolean logic.
1. if
, elif
, and else
Statements
The if
, elif
, and else
statements are the core of control flow in Python. They allow you to execute certain pieces of code based on whether a condition is true or false.
if
Statement
The if
statement evaluates a condition (an expression that returns True
or False
). If the condition is True
, the indented block of code following the if
statement is executed. If the condition is False
, the block of code is skipped.

In this example, since x
is indeed greater than 5, the message “x is greater than 5” is printed.
elif
Statement
The elif
(short for “else if”) statement allows you to check multiple conditions sequentially. If the first if
condition is False
, Python will check the next elif
condition, and so on. If an elif
condition is True
, its corresponding block of code is executed, and the rest of the elif
and else
blocks are skipped.

Here, since x
is not greater than 15 but is greater than 5, the second message is printed.
else
Statement
The else
statement provides a block of code that will be executed if none of the preceding if
or elif
conditions are True
.

Since x
is not greater than 5, the else
block is executed, and “x is 5 or less” is printed.
2. Nested Conditions
You can nest if
, elif
, and else
statements inside one another to create more complex decision-making structures.
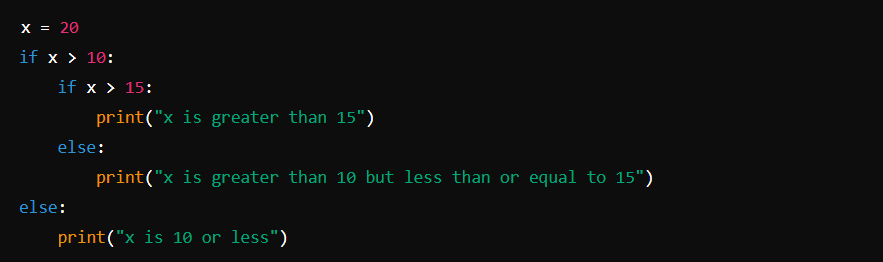
In this example:
- The outer
if
condition checks ifx
is greater than 10. - Since
x
is 20, the condition isTrue
, and the innerif
condition is evaluated. - The inner
if
condition checks ifx
is greater than 15, which is alsoTrue
, so “x is greater than 15” is printed.
3. Boolean Logic
Boolean logic involves combining multiple conditions using logical operators: and
, or
, and not
.
and
Operator
The and
operator returns True
if both conditions are True
.

Here, both conditions are True
, so “Both conditions are true” is printed.
or
Operator
The or
operator returns True
if at least one of the conditions is True
.

In this case, the first condition is True
, so “At least one condition is true” is printed.
not
Operator
The not
operator inverts the value of a condition.

Since x
is not greater than 15, the condition not x > 15
is True
, and “x is not greater than 15” is printed.
Combining Boolean Logic with Control Flow
You can combine Boolean logic with if
, elif
, and else
statements to create complex control flow.
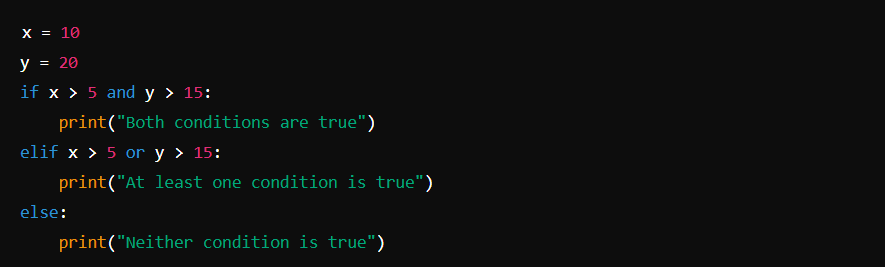
In this example:
- The first
if
condition isTrue
, so “Both conditions are true” is printed. - The
elif
andelse
blocks are skipped because the first condition wasTrue
.
Summary
Control flow is essential for making decisions in your Python programs. Understanding how to use if
, elif
, and else
statements, nested conditions, and Boolean logic allows you to build sophisticated and dynamic programs. Practice these concepts to become proficient in directing the flow of your code based on various conditions.