Strings are a fundamental data type in Python used to represent text. This tutorial will guide you through the various ways to work with strings, including string creation, manipulation, and common operations.
Creating Strings
In Python, you can create strings using single quotes ('
), double quotes ("
), triple single quotes ('''
), or triple double quotes ("""
).
Example: Creating Strings
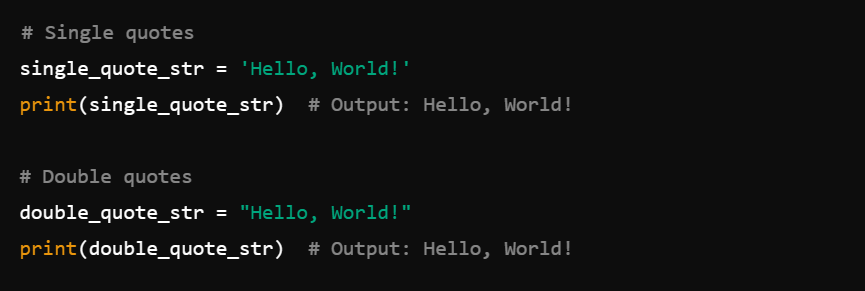
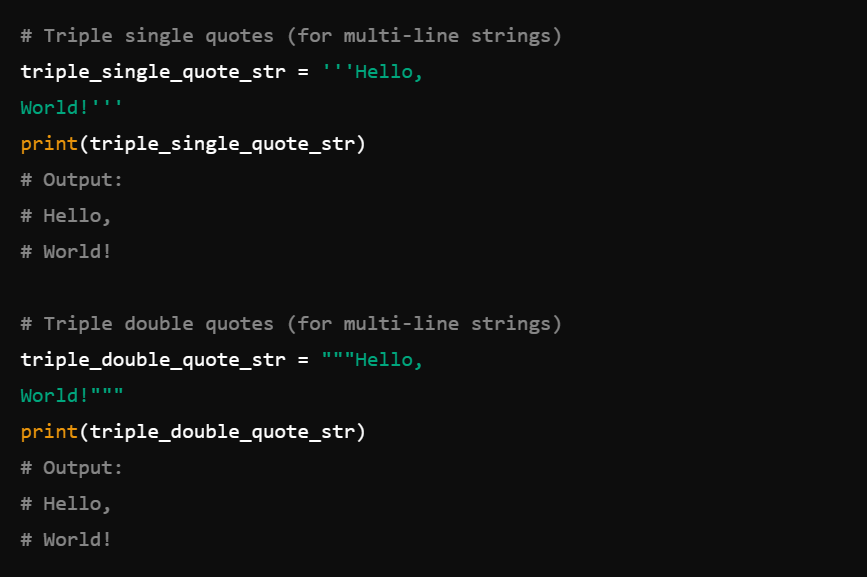
String Operations
1. Concatenation
You can concatenate (join) strings using the +
operator.
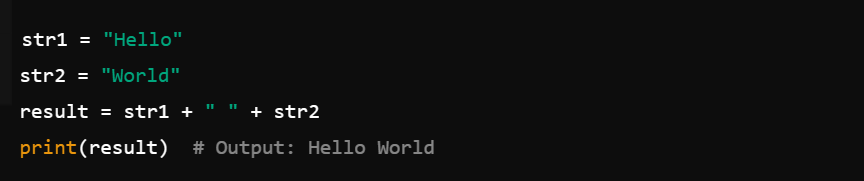
2. Repetition
You can repeat strings using the *
operator.

3. Indexing
You can access individual characters in a string using indexing. Indexes start from 0.

4. Slicing
You can access a range of characters using slicing. The syntax is string[start:end]
.
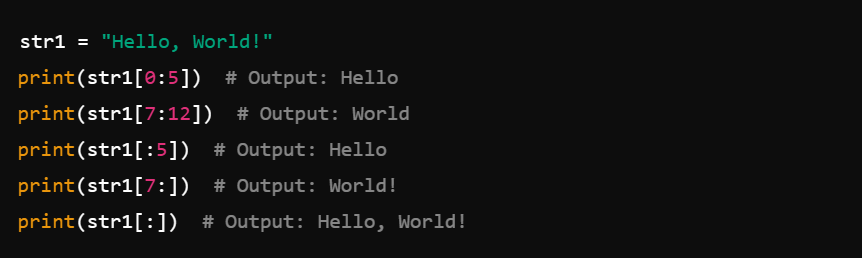
5. Length
You can find the length of a string using the len()
function.

String Methods
Python provides many built-in methods to manipulate strings.
1. upper()
Converts all characters to uppercase.

2. lower()
Converts all characters to lowercase.

3. strip()
Removes leading and trailing whitespace.

4. replace()
Replaces all occurrences of a substring with another substring.

5. split()
Splits a string into a list of substrings based on a delimiter.

6. join()
Joins a list of strings into a single string with a specified delimiter.

String Formatting
Python provides several ways to format strings, including the %
operator, the format()
method, and f-strings (formatted string literals).
1. %
Operator
Old style string formatting using the %
operator.
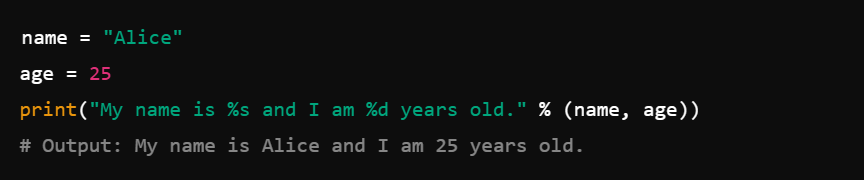
2. format()
Method
String formatting using the format()
method.
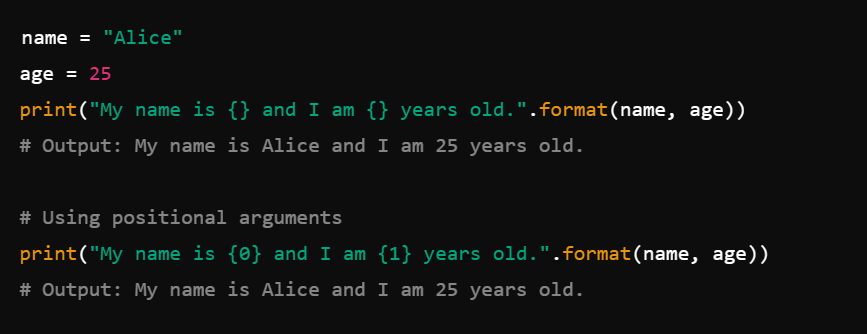
3. F-strings
String formatting using f-strings (available in Python 3.6+).

Escape Characters
Escape characters are used to represent special characters within strings. The backslash (\
) is used as an escape character.
Example: Escape Characters
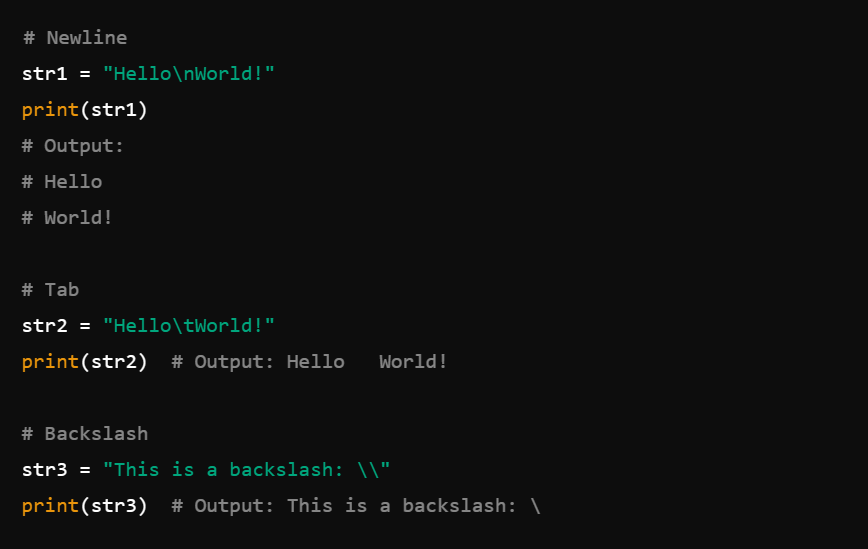
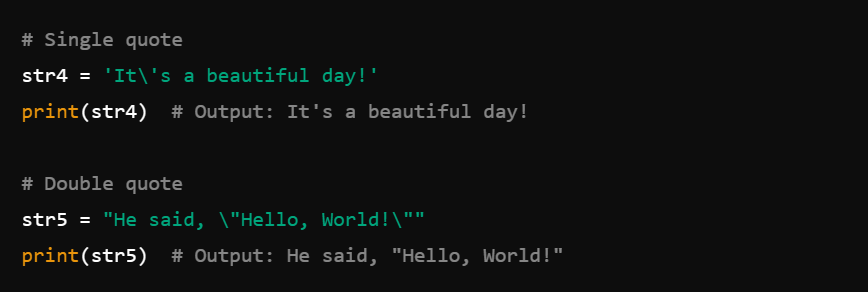
Multiline Strings
You can create multiline strings using triple quotes ('''
or """
).
Example: Multiline Strings
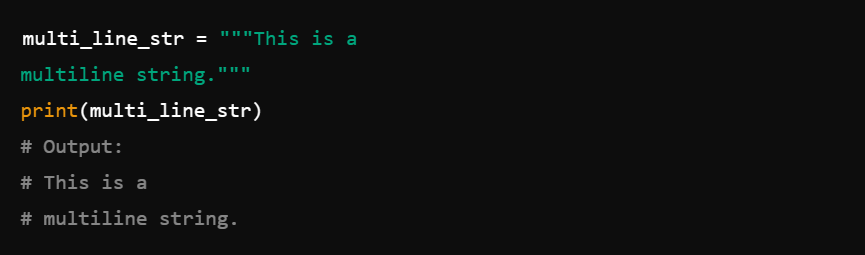
In this tutorial, we covered the basics of working with strings in Python. We learned how to create strings, perform various operations, use string methods, format strings, and handle escape characters. Understanding how to work with strings is essential for effective programming in Python. Practice these concepts by writing your own code and experimenting with different string operations and methods.