Variables and data types are foundational concepts in programming. Variables store data, and data types define the type of data a variable can hold. In this tutorial, we’ll focus on the four basic data types in Python: integers, floats, strings, and booleans.
Variables
A variable is a named location in memory that stores a value. In Python, you don’t need to declare the variable type explicitly. You can assign a value to a variable using the assignment operator (=
).
Example: Variable Assignment
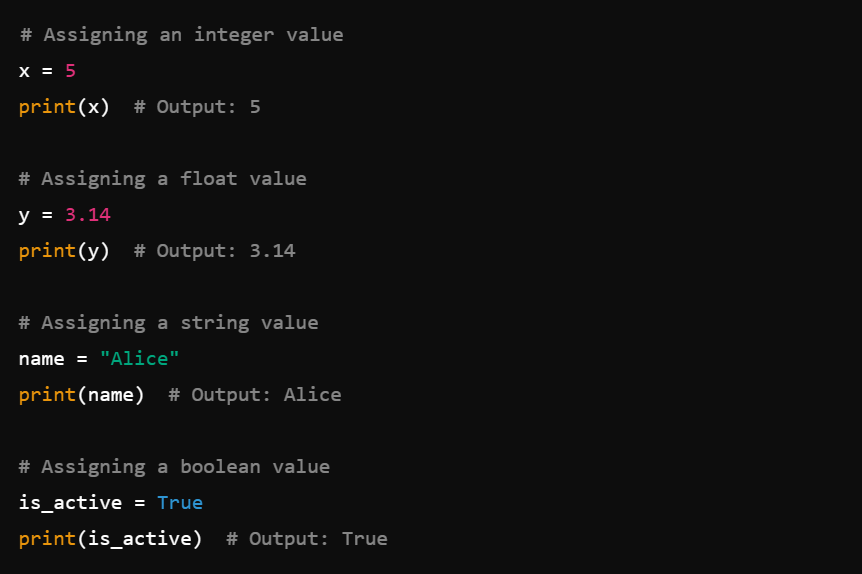
Variable Naming Rules
- Variable names must start with a letter or an underscore (
_
). - The rest of the name can contain letters, numbers, or underscores.
- Variable names are case-sensitive (
myVar
andmyvar
are different). - Avoid using Python keywords (e.g.,
if
,else
,while
, etc.) as variable names.
Example: Valid and Invalid Variable Names
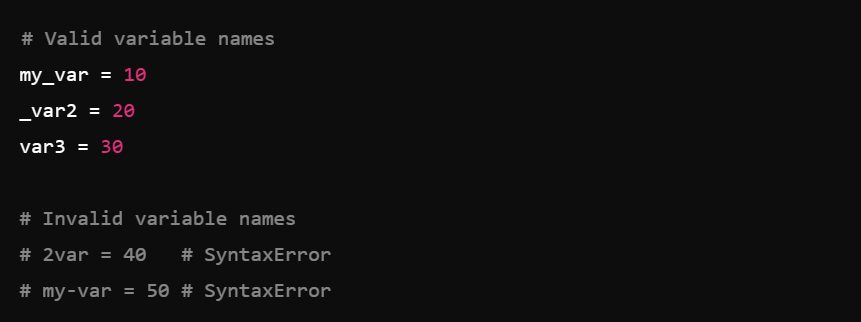
Basic Data Types
Python supports several basic data types:
- Integer (
int
) - Float (
float
) - String (
str
) - Boolean (
bool
)
1. Integer (int
)
Integers are whole numbers without a decimal point.

2. Float (float
)
Floats are numbers with a decimal point.

3. String (str
)
Strings are sequences of characters enclosed in single or double quotes.

4. Boolean (bool
)
Booleans represent one of two values: True
or False
.

Type Conversion
You can convert between different data types using built-in functions like int()
, float()
, str()
, and bool()
.
Example: Type Conversion

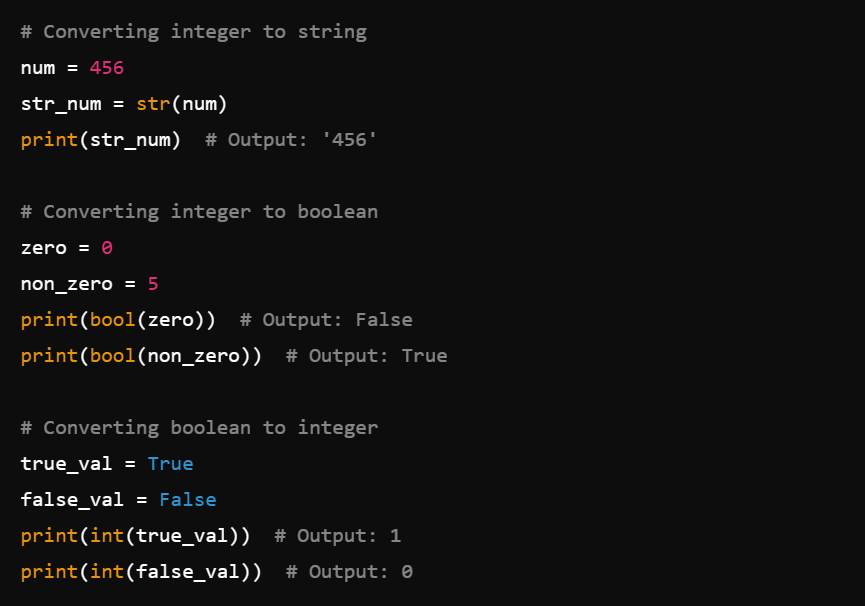
Checking Data Types
You can check the type of a variable using the type()
function.
Example: Checking Data Types
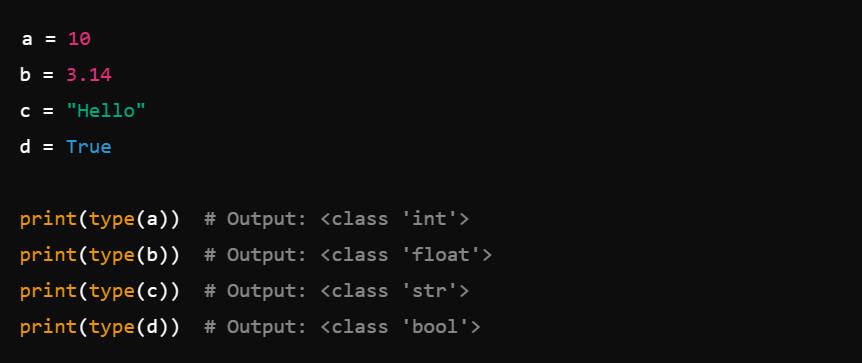
In this tutorial, we covered variables and the four basic data types in Python: integers, floats, strings, and booleans. We learned how to create variables, the rules for naming them, and how to perform operations on different data types. Understanding variables and basic data types is crucial for writing effective Python programs. Practice these concepts by writing your own code and experimenting with different data types and operations.