Exception handling is a critical part of programming in Python. It allows developers to anticipate and manage errors gracefully, ensuring that the program can continue running or fail in a controlled manner. In this tutorial, we’ll cover the basics of exception handling in Python, including try
, except
, else
, and finally
blocks, and we’ll also explore how to create and use custom exceptions.
Table of Contents
- Introduction to Exceptions
- The
try
andexcept
Blocks - The
else
Block - The
finally
Block - Catching Multiple Exceptions
- Best Practices for Exception Handling
- Creating and Using Custom Exceptions
- Examples and Use Cases
1. Introduction to Exceptions
In Python, an exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. When an error occurs within a program, Python raises an exception. If the exception is not handled, the program will terminate and display an error message.
Common built-in exceptions include:
ZeroDivisionError
: Raised when a division by zero occurs.ValueError
: Raised when a function receives an argument of the correct type but an inappropriate value.TypeError
: Raised when an operation or function is applied to an object of inappropriate type.FileNotFoundError
: Raised when trying to open a file that does not exist.
2. The try
and except
Blocks
The try
block lets you test a block of code for errors. The except
block lets you handle the error. You can have multiple except
blocks to handle different exceptions.
Syntax

Example

3. The else
Block
The else
block is optional and is used to execute code if no exceptions are raised in the try
block.
Syntax
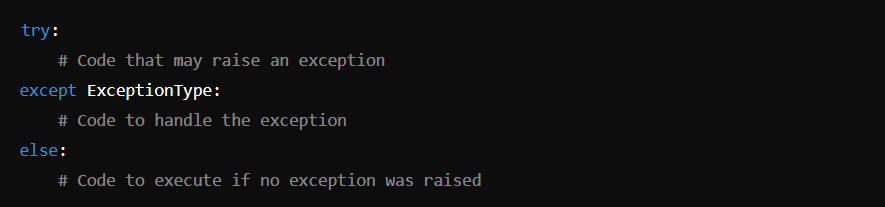
Example
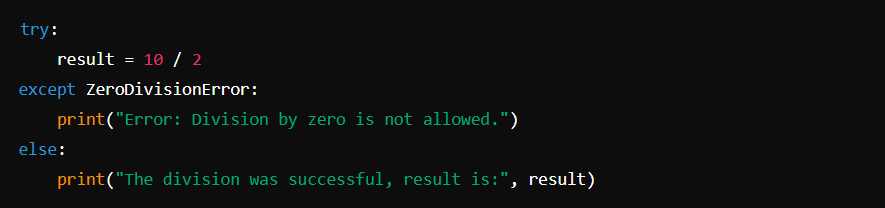
4. The finally
Block
The finally
block is optional and is used to execute code, regardless of whether an exception was raised or not. It is often used for cleanup actions (e.g., closing files or releasing resources).
Syntax
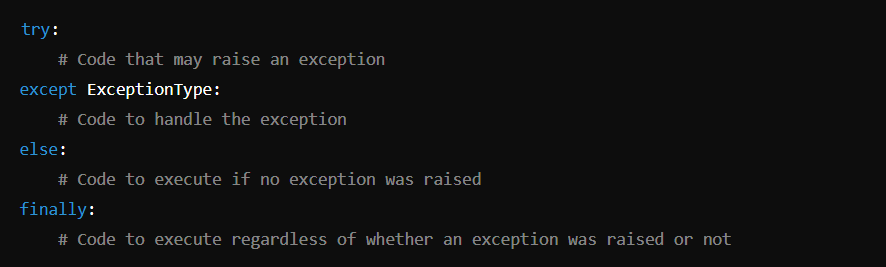
Example
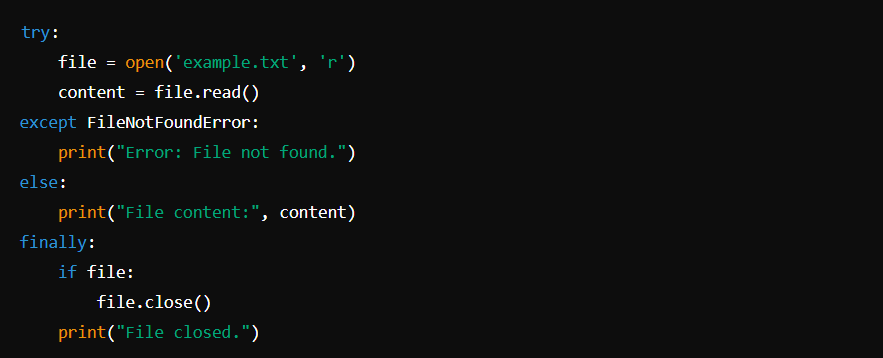
5. Catching Multiple Exceptions
You can catch multiple exceptions by specifying multiple except
blocks. Alternatively, you can catch multiple exceptions in a single except
block using a tuple.
Example with Multiple except
Blocks

Example with a Tuple

6. Best Practices for Exception Handling
- Be Specific: Catch specific exceptions rather than a general
Exception
to handle only the errors you expect. - Keep It Simple: Handle exceptions in a way that makes the code easy to read and maintain.
- Use
finally
for Cleanup: Ensure that resources are released properly, even if an error occurs. - Avoid Silent Failures: Always handle exceptions in a way that provides meaningful feedback to the user or logs the error for debugging.
7. Creating and Using Custom Exceptions
In addition to built-in exceptions, you can create custom exceptions to handle specific error conditions in your program.
Creating a Custom Exception
Custom exceptions should inherit from the base Exception
class or one of its subclasses.
Example
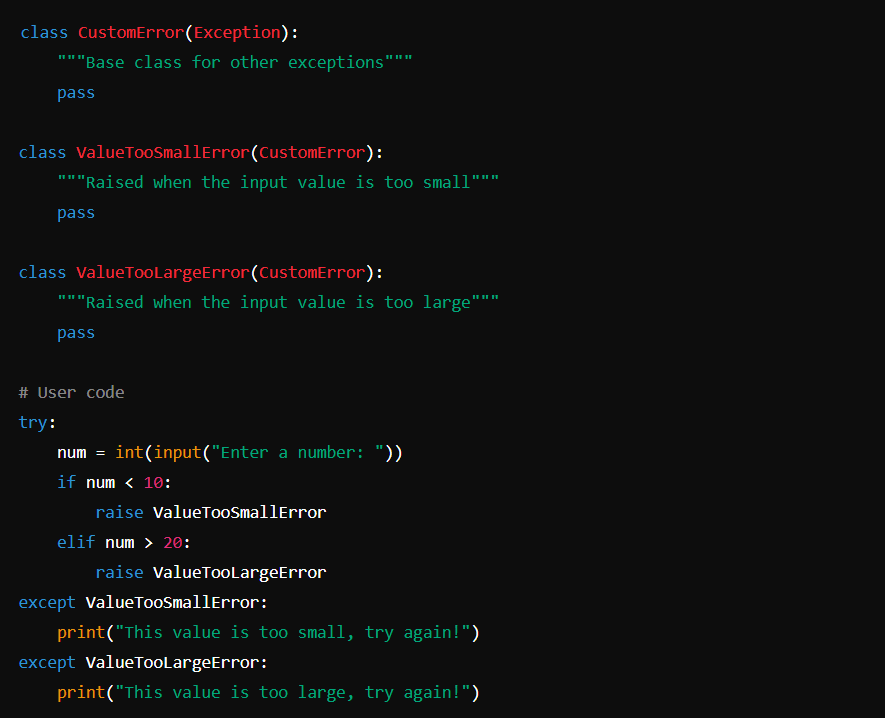
Using Custom Exceptions in Functions
Custom exceptions can be used in functions to signal specific error conditions.
Example
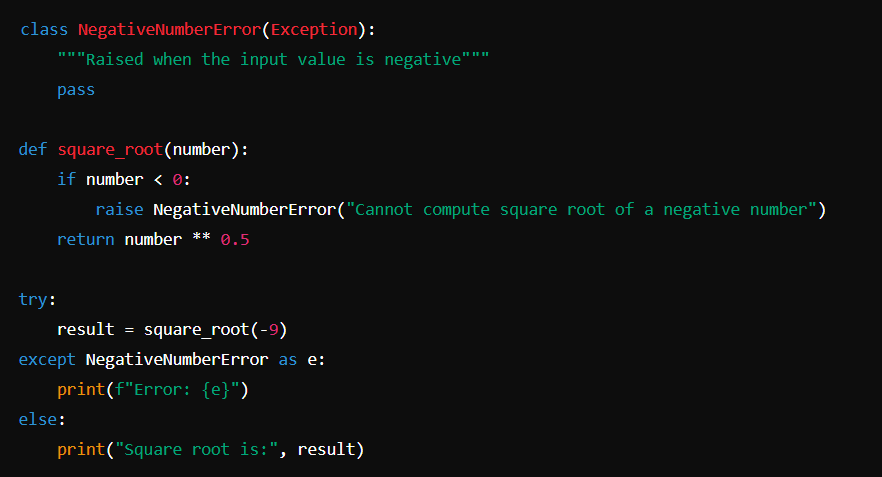
8. Examples and Use Cases
Example 1: Handling File Operations
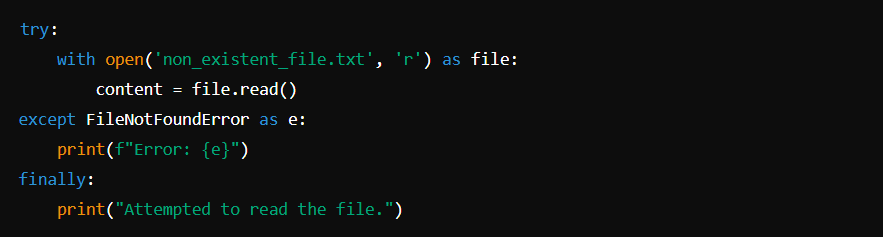
Example 2: Handling User Input
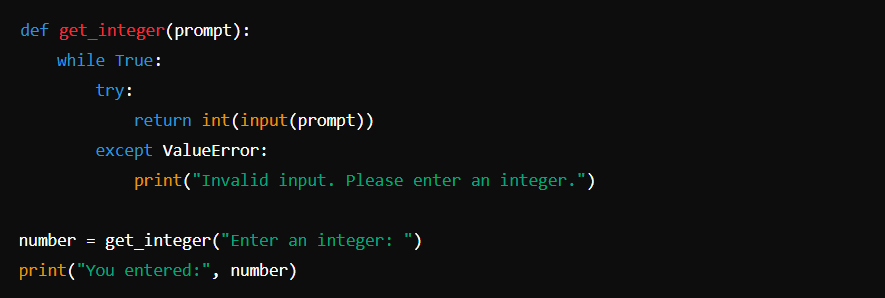
Example 3: Network Operations
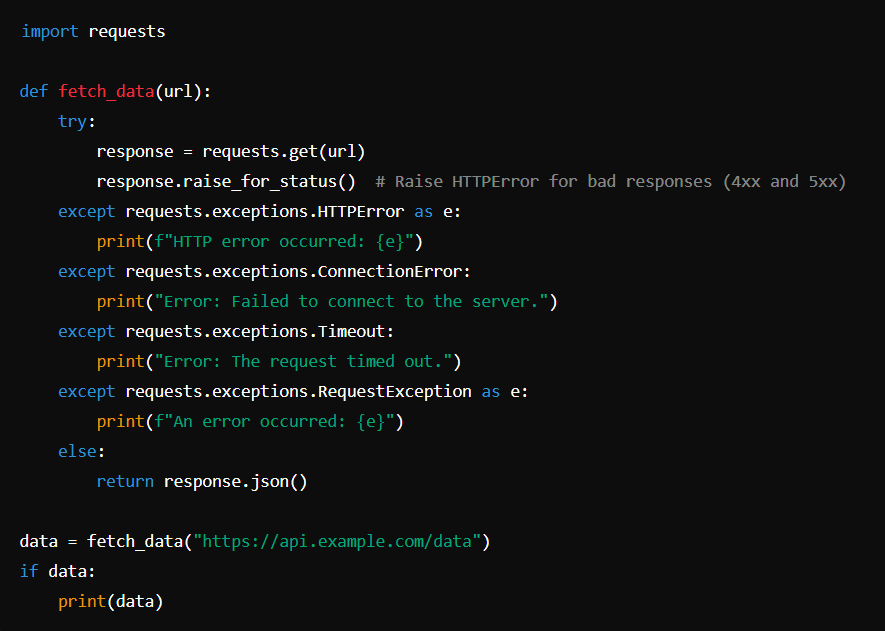
Example 4: Using Custom Exceptions
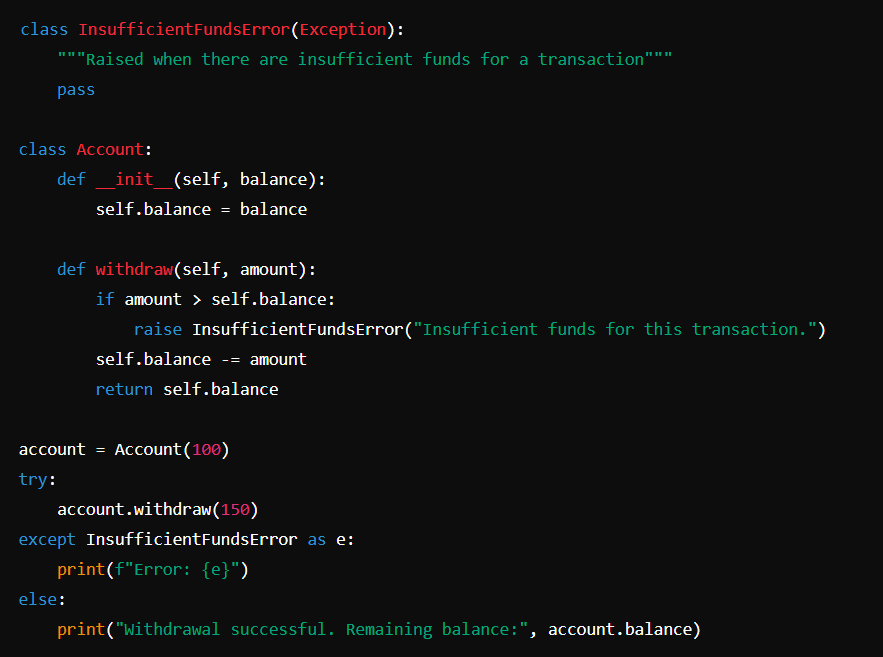
Conclusion
Exception handling is a powerful feature in Python that helps you build robust and resilient applications. By understanding and using try
, except
, else
, and finally
blocks effectively, and by creating custom exceptions when necessary, you can manage errors gracefully and provide a better user experience. Always strive to write clear and maintainable exception handling code, following best practices to ensure that your applications are both reliable and easy to debug.