Loops are essential in programming as they allow you to execute a block of code repeatedly. This tutorial covers the two main types of loops in Python—for
loops and while
loops—along with the break
and continue
statements that control the flow within these loops.
1. for
Loops
A for
loop is used to iterate over a sequence (e.g., a list, tuple, dictionary, set, or string) and execute a block of code for each item in the sequence.
Basic Syntax

Example with a List

This loop will print each number in the list numbers
.
Example with a String

This loop will print each character in the string message
.
Using range()
The range()
function generates a sequence of numbers, which is particularly useful in for
loops.

This loop will print numbers from 0 to 4.
2. while
Loops
A while
loop repeatedly executes a block of code as long as a given condition is True
.
Basic Syntax

Example

This loop will print numbers from 0 to 4 and then stop because the condition count < 5
becomes False
.
3. break
and continue
Statements
These statements provide additional control over the loop’s execution.
break
Statement
The break
statement is used to exit the loop immediately, regardless of the loop’s condition.
Example in a for
Loop

This loop will print numbers from 0 to 4 and then exit when i
equals 5.
Example in a while
Loop
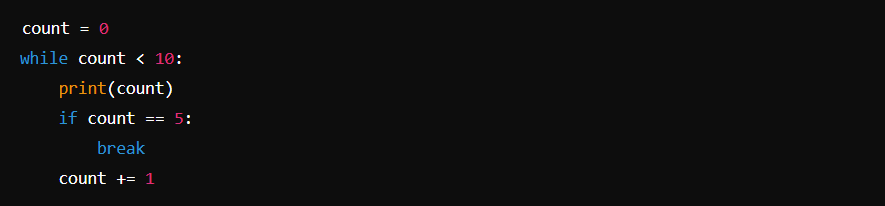
This loop will print numbers from 0 to 5 and then exit.
continue
Statement
The continue
statement skips the rest of the code inside the loop for the current iteration and moves to the next iteration.
Example in a for
Loop

This loop will print only odd numbers between 0 and 9.
Example in a while
Loop
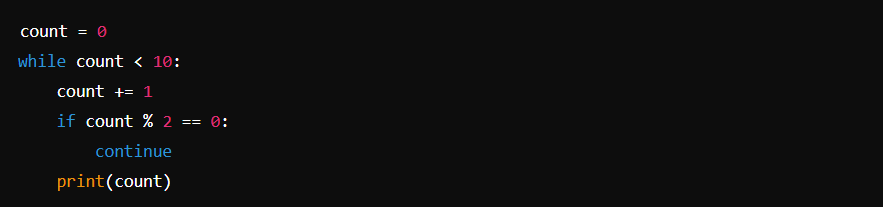
This loop will print only odd numbers from 1 to 9.
4. Nested Loops
You can nest loops inside other loops. This is often used for working with multi-dimensional data structures, like lists of lists.
Example with Nested for
Loops
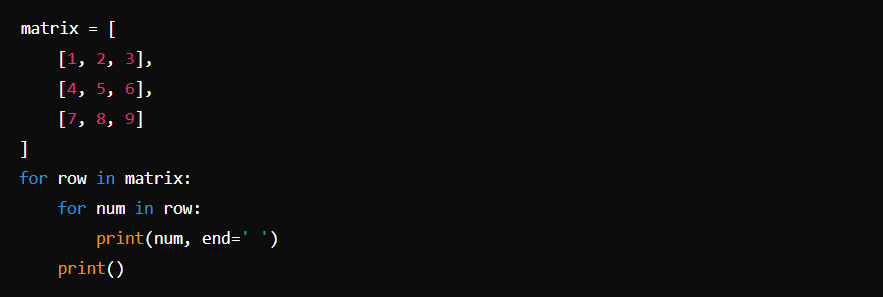
This will print each element in the matrix in a structured format.
5. Using else
with Loops
Python allows you to use an else
clause with loops. The else
block is executed only if the loop completes normally (i.e., not terminated by a break
statement).
Example with a for
Loop

This will print numbers from 0 to 4 and then print “Loop completed”.
Example with a while
Loop
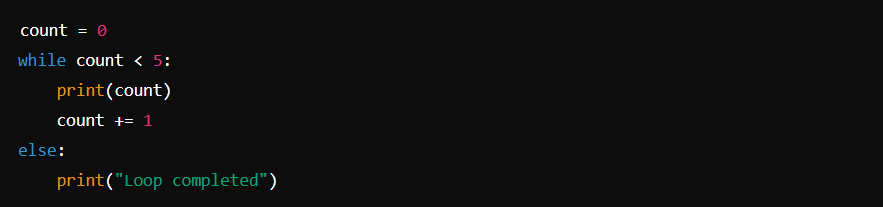
This will print numbers from 0 to 4 and then print “Loop completed”.
Summary
for
Loops: Ideal for iterating over a sequence.while
Loops: Ideal for repeating a block of code as long as a condition isTrue
.break
Statement: Exits the loop immediately.continue
Statement: Skips the rest of the code in the current iteration and moves to the next iteration.- Nested Loops: Loops inside other loops for multi-dimensional data.
else
with Loops: Executes a block of code if the loop completes normally.
Mastering these concepts will enable you to handle repetitive tasks efficiently and make your code more dynamic and powerful.